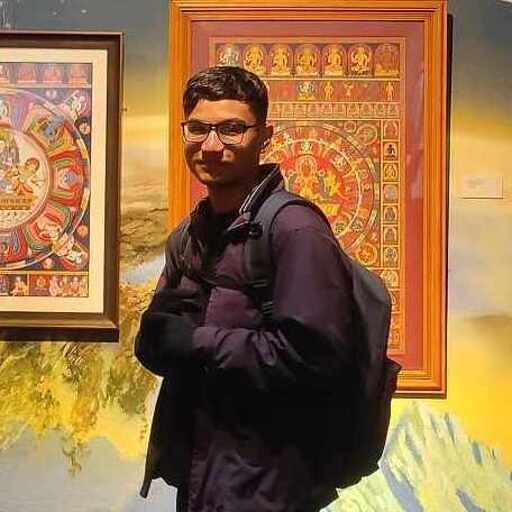
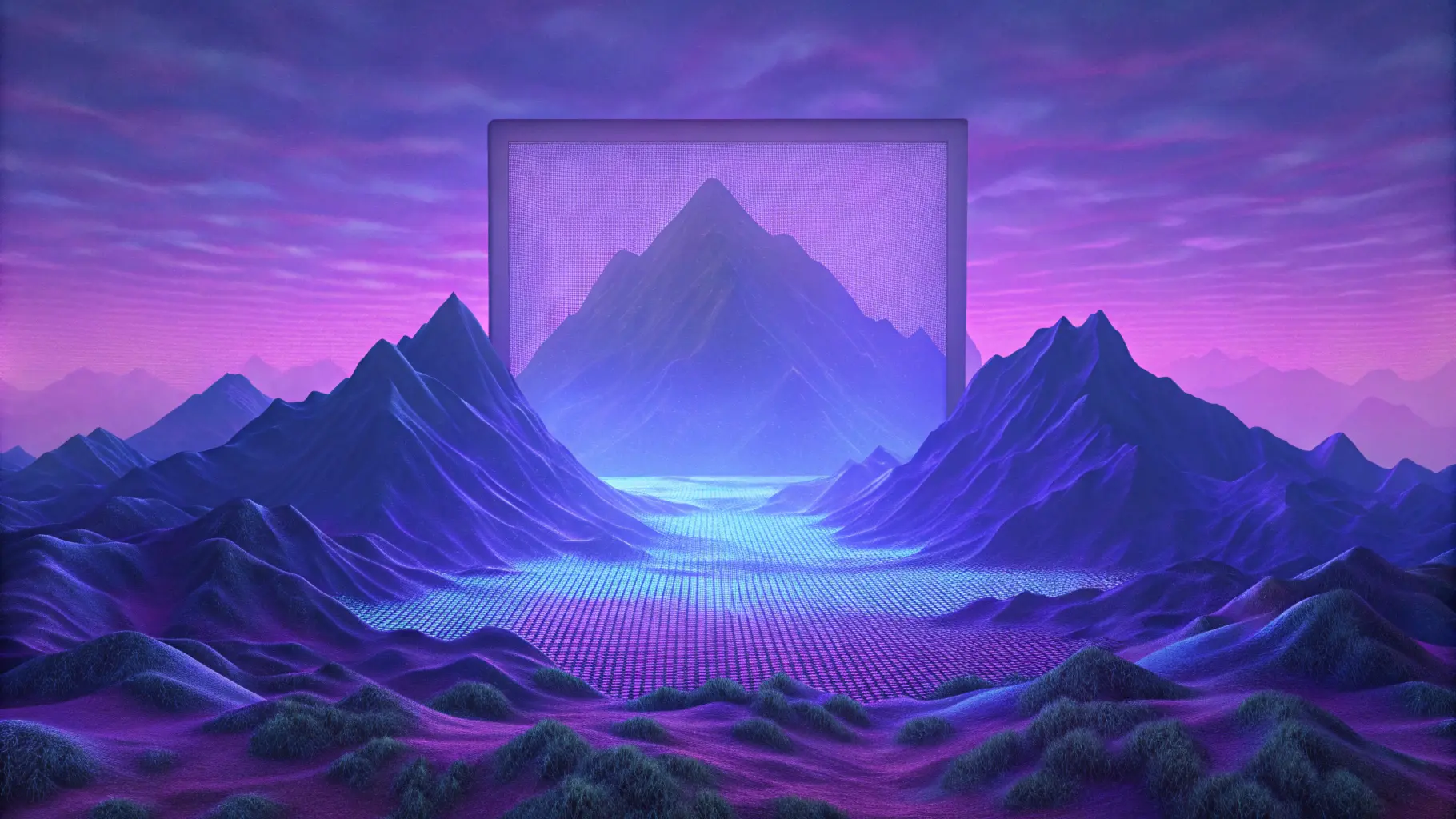
Background blurring is essential in enhancing privacy in virtual meetings and video recordings by obscuring sensitive surroundings. It allows creators and photographers to improve content quality by directing attention to the subject. Background blurring has become a key feature in popular tools like Google Meet and CapCut, streamlining video conferencing and editing.
In this tutorial, I'll guide you through building a background blurring tool that works seamlessly with both videos and images.
Use Cases of the Background Blurring Tool
- Hides sensitive or cluttered surroundings
- Directs attention to the subject
- Enhances appearance in virtual meetings
- Simplifies the background for real-time video editing
Building Your Background Blurring Tool
This tool uses two main components:
- A background removal model to create masks
- FFmpeg to apply the blur effect
Let's build it step by step.
Initial Setup
First, sign up for Sieve and get your API key. Then install and authenticate:
pip install sievedata
sieve login
Once you've logged in, our Background Blurring tool should be able to perform the following two key steps:
Step 1: Create a Raw Mask of the Media
We will use the sieve/background-removal function to generate a raw mask, where the resulting media will have a black background and the foreground subject will be white.
import sieve
# Generate raw mask using background removal model
file = sieve.File("your_file_url_or_path")
background_removal = sieve.function.get("sieve-internal/background-removal")
output = background_removal.push(
input_file=file,
yield_output_batches=False,
output_type="raw_mask"
)
output_object = next(output.result())
masked_video = sieve.File(path=output_object.path)
Example of a Raw Mask
Step 2: Utilize the Raw Mask to blur the background
Next, we'll use ffmpeg to apply the raw mask, blurring the background while preserving the foreground. The ffmpeg command will differ slightly depending on whether the media is an image or a video.
from pathlib import Path
import subprocess
# Configure blur strength and detect media type
SIGMA = 20 # strength of blur
is_image = file.path.lower().endswith(('.jpeg', '.jpg', '.png', '.bmp', '.tiff', '.webp'))
# Build ffmpeg command
command = [
"ffmpeg", "-y",
"-i", file.path, # original
"-i", masked_video.path, # raw mask
"-filter_complex",
f"[0:v]gblur=sigma={SIGMA}[blurred];[1:v]format=gray[mask];[blurred][0:v][mask]maskedmerge[out]",
"-map", "[out]"
]
# Add format-specific settings
if is_image:
command.extend(["-c:v", "png"])
else:
command.extend([
"-c:v", "libx264",
"-crf", "23",
"-preset", "fast",
"-map", "0:a?",
"-c:a", "copy"
])
# Set output filename and run command
extension = Path(file.path).suffix
command.append(f"output{extension}")
subprocess.run(command, check=True)
Example Outputs
Here are some before/after examples demonstrating the tool's capabilities:
Advanced Features
To enhance your blurring tool, you could add:
- Selective Region Blurring: Target specific areas only
- Real-Time Processing: Enable live video blurring
- GPU Acceleration: Optimize performance
- Motion Blur Effects: Add dynamic blur for movement
Conclusion
You now have a robust background blurring tool powered by Sieve's background-removal pipeline. This approach can be integrated into any video processing pipeline that needs high-quality background manipulation.