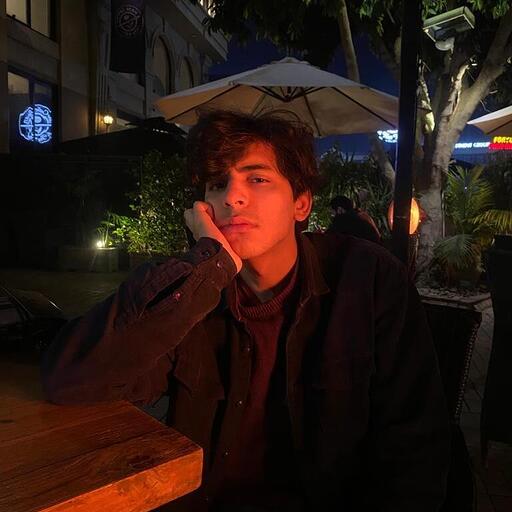
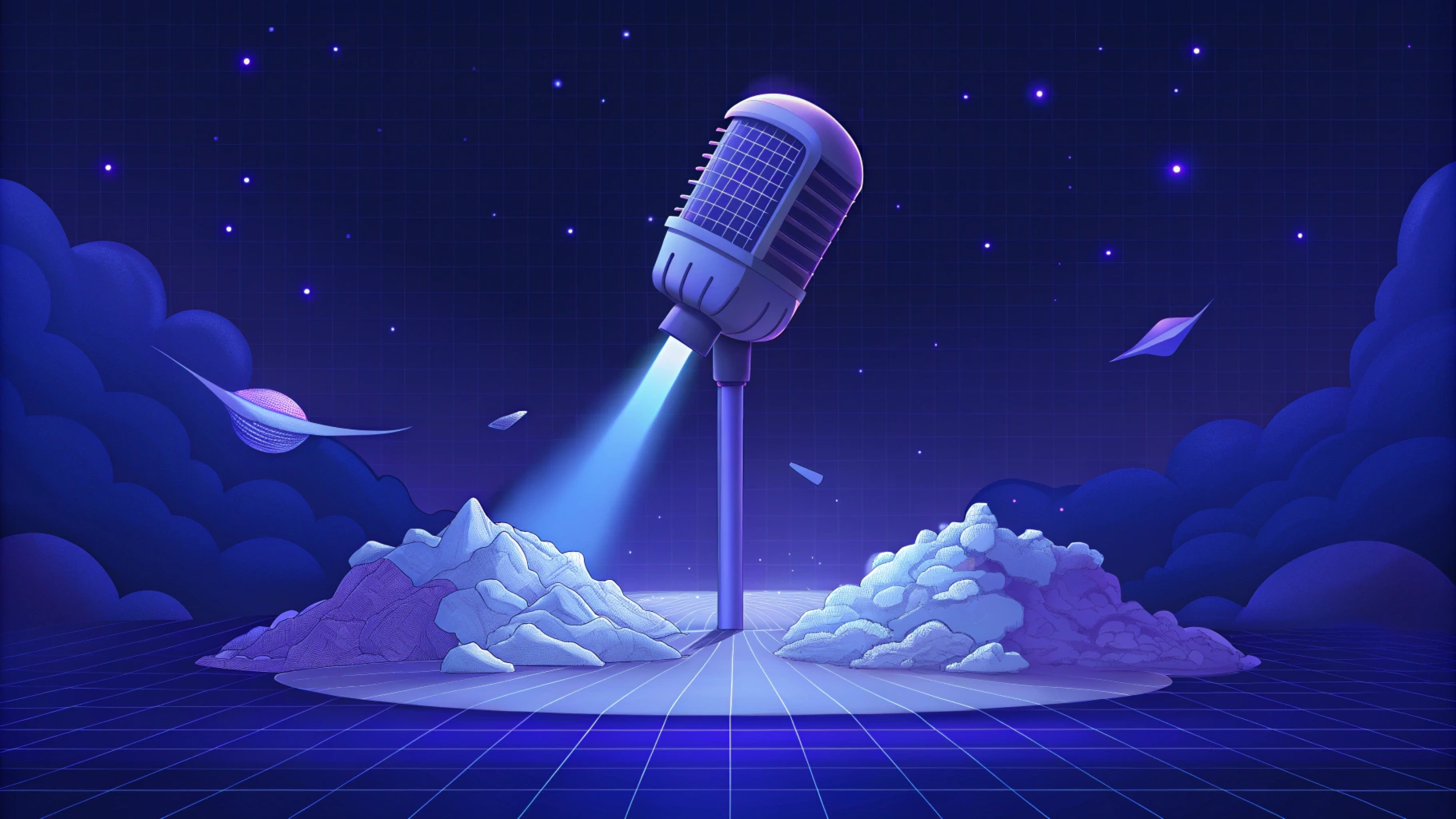
Video background noise removal is a critical requirement for developers working with audio-visual content like meetings, tutorials, and social media videos. Clean, noise-free audio directly impacts viewer engagement and content quality. This technical guide explores different programmatic approaches to video background noise removal and provides implementation examples for each method.
Traditional DSP Approaches to Background Noise Removal
Traditional digital signal processing (DSP) techniques offer lightweight solutions for video background noise removal without requiring AI models. These methods include spectral subtraction for noise estimation and removal, noise gates for ambient sound reduction, and adaptive filters for real-time processing. While simpler than deep learning approaches, they can be effective for basic noise removal tasks.
Pros
- Simple Implementation: Easy to set up without needing complex models or training.
- Low Computational Cost: Efficient and works well in resource-limited environments.
- Real-Time Processing: Suitable for live video applications.
- Adjustable Parameters: Can be customized to fit specific noise characteristics.
Cons
- Limited Effectiveness: Not ideal for complex or rapidly changing noise.
- Artifacts and Distortions: May introduce unwanted audio artifacts.
- Requires Tuning: Needs parameter adjustments for optimal results.
- Basic Performance: Often insufficient for professional audio quality.
The code below is an example of how one might implement this in Python.
from pydub import AudioSegment
import numpy as np
import scipy.io.wavfile as wav
from scipy.fftpack import fft, ifft
# Load the audio file
audio = AudioSegment.from_file("input_audio.wav")
audio_samples = np.array(audio.get_array_of_samples())
# Apply Fourier Transform to convert to frequency domain
spectrum = fft(audio_samples)
# Estimate the noise profile (assuming the first 0.5 seconds is noise)
noise_profile = np.mean(np.abs(spectrum[:int(0.5 * audio.frame_rate)]))
# Apply spectral subtraction
clean_spectrum = np.abs(spectrum) - noise_profile
clean_spectrum[clean_spectrum < 0] = 0 # Ensure no negative values
clean_audio_samples = ifft(clean_spectrum).real
# Convert back to audio and save
clean_audio = audio._spawn(clean_audio_samples.astype(np.int16).tobytes())
clean_audio.export("output_clean_audio.wav", format="wav")
print("Background noise removed and saved as 'output_clean_audio.wav'.")
Noise suppression models
Noise reduction models use deep learning to remove background noise while preserving voice clarity. Popular open-source models like Resemble Enhance and DeepFilterNet offer robust noise suppression, capable of handling complex and dynamic noise environments with greater precision than traditional DSP methods. These models are trained on large datasets of paired noisy and clean audio samples to learn how to map corrupted audio to its clean counterpart, allowing them to generalize to new types of noise and acoustic environments.
Pros
- High Effectiveness: Excellent at reducing noise while maintaining voice clarity.
- Handles Complex Noise: Works well in dynamic and unpredictable audio environments.
- Minimal Artifacts: Advanced models produce fewer distortions compared to traditional methods.
- Open-Source Availability: Easy access to robust models like Resemble Enhance and DeepFilterNet.
Cons
- Computationally Intensive: Requires more processing power, especially for real-time use.
- Dependency on Model Quality: Results vary based on the quality and optimization of the chosen model.
Using Sieve’s noise removal API
sieve/audio-enhance
is a useful pipeline for developers looking for access to the best audio enhancement and background noise removal models available. You can pick between models like Auphonic, ai|coustics, Cleanvoice, ElevenLabs, and Resemble Enhance and run it via Python (just pip install sievedata
) like below or via API.
import sieve
enhance = sieve.function.get("sieve/audio-enhance")
some_file = sieve.File("path/to/file")
output = enhance.run(some_file, task="denoise")
print("Saved to", output.path)
Source separation models
Source separation models use deep learning to isolate different audio components, such as voices and background sounds, from a mixed signal. While noise reduction models focus on suppressing noise while preserving the main audio, source separation models go further by disentangling the sources entirely, allowing for precise isolation of speech. Tools like Demucs and Spleeter excel in separating speech from background noise, making them highly effective for complex and dynamic audio environments. These models provide an alternative to noise reduction models, offering fine-grained control over audio components for cleaner results.
Pros
- Precise Isolation: Separates individual audio sources, providing clear voice isolation.
- Adaptability: Handles complex and layered audio environments effectively.
- High Customization: Offers fine-grained control over different audio components.
- Open-Source Tools: Access to powerful models like Demucs and Spleeter.
Cons
- High Resource Demand: Computationally heavy, requiring significant processing power.
- Processing Time: Longer processing compared to simpler noise reduction models.
- Potential Over-Isolation: May sometimes over-isolate, impacting natural audio quality.
Using Sieve’s Demucs API
sieve/demucs
is a useful hosted API for developers looking for access to state-of-the-art source separation techniques. You can run it via Python like below or via API.
import sieve
demucs = sieve.function.get("sieve/demucs")
some_file = sieve.File("path/to/file")
output = demucs.run(some_file)
print(output)
Conclusion
Background noise removal is crucial for clear audio, and developers have various tools at their disposal, from traditional DSP techniques to advanced deep learning models like noise reduction and source separation. By choosing the right approach for your needs, you can greatly enhance audio quality and deliver a more professional sound experience. Sieve gives you access to all these tools in one place, and you can get started with an account here.